Bagaimana cara mempercantik alert pada javascript?
Jawabannya adalah: ada banyak. Kita bisa membuat sendiri, atau menggunakan modal dari bootstrap, dan banyak sekali.
Tetapi, dalam kesempatan kali ini, kita akan menggunakan sweet alert js.
Contoh tampilan alert bawaan dari js:
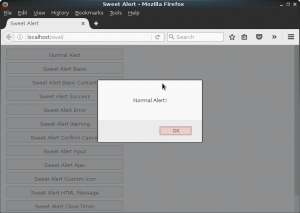
Normal Alert Javascript
Dan perhatikan bagaimana alert dengan sweet alert
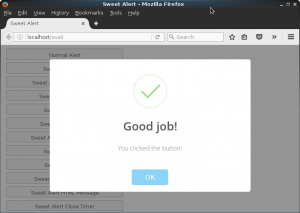
Sweet Alert Example
Langsung saja kita bisa menginstall sweet alert dengan 3 cara
- Melalui bower
$ bower install sweetalert - Melalui npm
$ npm install sweetalert - Manual / Download
- Download file master di https://github.com/t4t5/sweetalert/archive/master.zip
- Extract file hasil download
- Taruh file hasil extract ke folder project
Contoh Program:
<!DOCTYPE html> <html> <head> <title>Sweet Alert</title> <link rel="stylesheet" type="text/css" href="node_modules/sweetalert/dist/sweetalert.css"> <!-- <link rel="stylesheet" type="text/css" href="node_modules/sweetalert/themes/twitter/twitter.css"> <link rel="stylesheet" type="text/css" href="node_modules/sweetalert/themes/google/google.css"> <link rel="stylesheet" type="text/css" href="node_modules/sweetalert/themes/facebook/facebook.css"> --> <script type="text/javascript" src="node_modules/sweetalert/dist/sweetalert.min.js"></script> <style type="text/css"> button.mybutton{ display: block; min-width: 300px; height: 30px; padding: 5px; margin: 5px; } </style> </head> <body> <button class="mybutton" onclick="normal()">Normal Alert</button> <button class="mybutton" onclick="basic()">Sweet Alert Basic</button> <button class="mybutton" onclick="basicContent()">Sweet Alert Basic Content</button> <button class="mybutton" onclick="sweet()">Sweet Alert Success</button> <button class="mybutton" onclick="error()">Sweet Alert Error</button> <button class="mybutton" onclick="warning()">Sweet Alert Warning</button> <button class="mybutton" onclick="confirmCancel()">Sweet Alert Confirm Cancel</button> <button class="mybutton" onclick="input()">Sweet Alert Input</button> <button class="mybutton" onclick="ajax()">Sweet Alert Ajax</button> <button class="mybutton" onclick="customIcon()">Sweet Alert Custom Icon</button> <button class="mybutton" onclick="htmlMessage()">Sweet Alert HTML Message</button> <button class="mybutton" onclick="closeTimer()">Sweet Alert Close Timer</button> <button class="mybutton" onclick="prompt()">Sweet Alert Prompt</button> <script> function normal() { alert("Normal Alert!"); } function sweet(){ swal("Good job!", "You clicked the button!", "success"); } function error(){ swal("Good job!", "You clicked the button!", "error"); } function basic(){ swal("Good job!"); } function basicContent(){ swal("Good job!", "You are awesome!"); } function warning(){ swal({ title: "Are you sure?", text: "You will not be able to recover this imaginary file!", type: "warning", showCancelButton: true, confirmButtonColor: "#DD6B55", confirmButtonText: "Yes, delete it!", closeOnConfirm: false, html: false }, function(){ swal("Deleted!", "Your imaginary file has been deleted.", "success"); }); } function ajax(){ swal({ title: 'Ajax request example', text: 'Submit to run ajax request', type: 'info', showCancelButton: true, closeOnConfirm: false, disableButtonsOnConfirm: true, confirmLoadingButtonColor: '#DD6B55' }, function(inputValue){ setTimeout(function() { swal('Ajax request finished!'); }, 2000); }); } function confirmCancel(){ swal({ title: "Are you sure?", text: "You will not be able to recover this imaginary file!", type: "warning", showCancelButton: true, confirmButtonColor: "#DD6B55", confirmButtonText: "Yes, delete it!", cancelButtonText: "No, cancel plx!", closeOnConfirm: false, closeOnCancel: false }, function(isConfirm){ if (isConfirm) { swal("Deleted!", "Your imaginary file has been deleted.", "success"); } else { swal("Cancelled", "Your imaginary file is safe :)", "error"); } }); } function customIcon(){ swal({ title: "Sweet!", text: "Here's a custom image.", imageUrl: "node_modules/sweetalert/example/images/thumbs-up.jpg" }); } function htmlMessage(){ swal({ title: "HTML <small>Title</small>!", text: 'A custom <span style="color:#F8BB86">html<span> message.', html: true }); } function closeTimer(){ swal({ title: "Auto close alert!", text: "I will close in 2 seconds.", timer: 2000, showConfirmButton: false }); } function prompt(){ swal({ title: "An input!", text: "Write something interesting:", type: "input", showCancelButton: true, closeOnConfirm: false, animation: "slide-from-top", inputPlaceholder: "Write something" }, function(inputValue){ if (inputValue === false) return false; if (inputValue === "") { swal.showInputError("You need to write something!"); return false } swal("Nice!", "You wrote: " + inputValue, "success"); }); } function input(){ swal({ title: "An input!", text: 'Write something interesting:', type: 'input', showCancelButton: true, closeOnConfirm: false, animation: "slide-from-top" }, function(inputValue){ swal("You wrote "+inputValue); }); } </script> </body> </html>
Untuk dokumentasi lebih lengkap bisa langsung ke alamat http://t4t5.github.io/sweetalert/